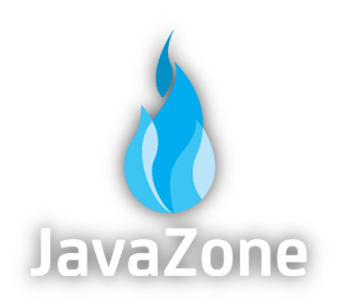
The client–server communication is further constrained by no client context being stored on the server between requests. Each request from any client contains all of the information necessary to service the request, and any session state is held in the client.
Authorization: AWS AWSAccessKeyId:Signature
Authorization = "AWS" + " " + AWSAccessKeyId + ":" + Signature; Signature = Base64( HMAC-SHA1( UTF-8-Encoding-Of( YourSecretAccessKeyID, StringToSign ) ) ); StringToSign = HTTP-Verb + "\n" + Content-MD5 + "\n" + Content-Type + "\n" + Date + "\n" + CanonicalizedAmzHeaders + CanonicalizedResource; CanonicalizedResource = [ "/" + Bucket ] + <HTTP-Request-URI, from the protocol name up to the query string> + [ sub-resource, if present. For example "?acl", "?location", "?logging", or "?torrent"]; CanonicalizedAmzHeaders = [lower cased, sorted, trimmed, joined with newline]
400 Yeah, I've heard that before...
<?xml version="1.0" encoding="utf-8"?> <!DOCTYPE foo [ <!ENTITY a "1234567890" > <!ENTITY b "&a;&a;&a;&a;&a;&a;&a;&a;" > <!ENTITY c "&b;&b;&b;&b;&b;&b;&b;&b;" > <!ENTITY d "&c;&c;&c;&c;&c;&c;&c;&c;" > <!ENTITY e "&d;&d;&d;&d;&d;&d;&d;&d;" > <!ENTITY f "&e;&e;&e;&e;&e;&e;&e;&e;" > <!ENTITY g "&f;&f;&f;&f;&f;&f;&f;&f;" > <!ENTITY h "&g;&g;&g;&g;&g;&g;&g;&g;" > <!ENTITY i "&h;&h;&h;&h;&h;&h;&h;&h;" > <!ENTITY j "&i;&i;&i;&i;&i;&i;&i;&i;" > <!ENTITY k "&j;&j;&j;&j;&j;&j;&j;&j;" > <!ENTITY l "&k;&k;&k;&k;&k;&k;&k;&k;" > <!ENTITY m "&l;&l;&l;&l;&l;&l;&l;&l;" > ]> <foo>&m;</foo>
<?xml version="1.0" encoding="utf-8"?> <foo> <foo> <foo> <foo> <foo> <foo> ...1 million foos later... <foo> Hola el mundo </foo> ...there and back again... </foo> </foo> </foo>
$(...).text(val)instead of
$(...).html(val)
X-Content-Type-Options: nosniff
$("body").bind("ajaxSend", function(elm, xhr, s){ if (s.type == "POST") { xhr.setRequestHeader('X-CSRF-Token', csrf_token); } });
X-Frame-Options: DENY
https://example.com/some/resource?id=2&signature=ab9829dfh02920920220baa422b6cc7
bar=2&baz=3&foo=1
SECRETbar2baz3foo1
bar=2&baz=3&foo=1&signature=afb12318a0b9823bcd
?bar=2&baz=3&foo=1and
?b=ar2baz3foo1
?b=ar2baz3foo10000020&bar=6&baz=5&foo=4and then length extend the signature to include the new parameters
@ResourceFilters({ AuthenticatedUserAdminFilter.class, CsrfVerificationFilter.class, NoCacheResponseFilter.class }) @Path(AccountResource.ACCOUNTS_BASE_PATH) public class BaseResource {